As a software engineer, there are several key areas of coding and concepts you should be comfortable with. The specific “important code” depends on the type of software you’re developing (e.g., web, mobile, systems, etc.), but here are the fundamental skills and coding topics that are crucial across the board:

1. Data Structures
Understanding data structures allows you to solve problems efficiently and is critical in interviews and real-world programming. You should know:
- Arrays and Lists: Basic structures for storing elements.
- Stacks and Queues: Useful for managing sequences and order of execution.
- Hash Maps / Hash Tables: For fast lookup and efficient key-value storage.
- Linked Lists: Important for dynamic data storage.
- Trees: Especially binary trees, AVL trees, and heaps for hierarchical data.
- Graphs: Representing networks or relationships (e.g., in social media, web crawling).
- Tries: Efficient for storing dictionaries and autocomplete.
2. Algorithms
Algorithms are essential to solving problems and optimizing solutions. You should know how to:
- Sorting: Merge sort, quicksort, bubble sort, insertion sort.
- Searching: Binary search, linear search.
- Graph algorithms: Dijkstra’s algorithm, breadth-first search (BFS), depth-first search (DFS).
- Dynamic Programming: Techniques like memoization and bottom-up approaches.
- Greedy Algorithms: For optimization problems (e.g., knapsack problem).
- Divide and Conquer: Problem-solving method useful for complex problems.
3. Object-Oriented Programming (OOP)
OOP is a core paradigm in many software engineering roles. It’s essential to know how to design and structure code:
- Classes and Objects: Building modular, reusable components.
- Inheritance: Reusing and extending functionality.
- Polymorphism: Changing behavior of objects based on context.
- Encapsulation: Hiding details of implementation.
- Abstraction: Simplifying complex systems by focusing on essential features.
4. Version Control (Git)
- Basic Git Commands: https://snaphub.host/wp-content/uploads/2025/02/coding.png
- Branching and Merging: Essential for collaboration and managing different features or bug fixes.
- Pull Requests / Code Reviews: For collaborative coding, version control helps manage contributions.
5. Database Management
Understanding databases and how to interact with them is a key skill:
- SQL (Structured Query Language): Writing queries, joins, subqueries, aggregation, and normalization.
- NoSQL Databases: Familiarity with key-value stores (e.g., Redis), document databases (e.g., MongoDB).
- Database Design: Understanding of normalization and indexing for efficient data storage and retrieval.
6. Web Development (for Web Software Engineers)
- HTML, CSS, and JavaScript: The building blocks of web development.
- Frameworks: React, Angular, Vue (front-end), Node.js, Django, Flask, Spring (back-end).
- RESTful APIs: Understanding of how to build and consume APIs.
- Authentication & Authorization: OAuth, JWT, session management.
7. Testing
- Unit Testing: Writing tests for small units of code to ensure correctness (e.g., using JUnit, PyTest, Mocha).
- Integration Testing: Ensuring different components of the system work together.
- Test-Driven Development (TDD): Writing tests before writing the code to guide development.
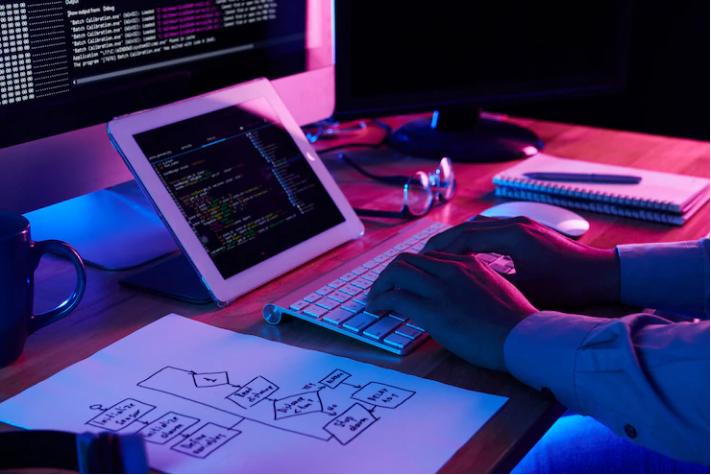
8. Concurrency and Multithreading
- Thread Management: Handling multiple threads to run code in parallel for efficiency (e.g., Java threads, Python’s
- Locks and Synchronization: Preventing data corruption when multiple threads access the same resource.
- Deadlock Prevention: Ensuring threads don’t get stuck waiting for each other indefinitely.
9. Design Patterns
Design patterns are reusable solutions to common problems:
- Singleton: Ensures only one instance of a class exists.
- Factory: Creates objects without specifying the exact class of object to be created.
- Observer: Allows an object to notify other objects when its state changes.
- Strategy: Allows algorithms to be selected at runtime.
- Decorator: Adds responsibilities to an object dynamically.
10. Cloud Computing & DevOps (Optional for certain roles)
- Cloud Platforms: Familiarity with AWS, Azure, or Google Cloud.
- Containerization: Docker for packaging applications and Kubernetes for orchestration.
- CI/CD: Continuous Integration and Continuous Deployment practices.
11. Security Basics
- Encryption & Decryption: Protecting sensitive data.
- SQL Injection Protection: Writing safe queries to prevent attacks.
- XSS & CSRF: Understanding and preventing cross-site scripting and cross-site request forgery.
12. Development Frameworks and Libraries
Depending on your specialization, learn the most popular tools:
- Front-End Frameworks: React, Vue.js, Angular.
- Back-End Frameworks: Django, Flask, Node.js, Spring.
- Mobile Development: Swift (iOS), Kotlin (Android), Flutter (cross-platform).
13. Basic Operating System Concepts
- File Systems: How the operating system handles files.
- Memory Management: Stack vs. heap, garbage collection, and memory leaks.
- Processes and Threads: How a program is executed and manages resources.
The best approach is to continuously practice these skills by building projects, solving problems, and collaborating with others. The more you work with these concepts, the more naturally they’ll come to you when you’re building software.